Deep Dive into Next.js Optimization Techniques: A Comprehensive Guide to Boosting Performance
Thursday, November 7, 2024
Understanding SSR vs. SSG
SSR (Server-Side Rendering)
SSR generates HTML dynamically on each request. While it's excellent for dynamic, real-time content, it can increase TTFB (Time to First Byte) if the server is slow.
SSG (Static Site Generation)
SSG generates HTML during build time. Ideal for content that doesn’t change often, it serves pre-rendered files, making it faster and more cacheable.
When to Use What?
- Use SSR for user-specific or frequently updated content (e.g., dashboards).
- Use SSG for static content like blogs or documentation.
Leveraging Incremental Static Regeneration (ISR)
ISR bridges the gap between SSR and SSG. It allows you to regenerate static pages at runtime, ensuring stale content is updated without rebuilding the entire site.
export async function getStaticProps() { const data = await fetchDataFromAPI(); return { props: { data }, revalidate: 60, // Revalidate page every 60 seconds }; }
Best Practices:
- Set a reasonable revalidate interval based on content update frequency.
- Use ISR for blogs, e-commerce product pages, or marketing sites.
Deep Dive into Next.js Optimization Techniques
Next.js has revolutionized modern web development with its seamless support for SSR (Server-Side Rendering), SSG (Static Site Generation), and CSR (Client-Side Rendering). While it provides great defaults, achieving peak performance requires a strategic approach. This guide explores advanced techniques to optimize Next.js applications for speed, scalability, and better user experience.
Understanding SSR vs. SSG
SSR (Server-Side Rendering)
SSR generates HTML dynamically on each request. While it's excellent for dynamic, real-time content, it can increase TTFB (Time to First Byte) if the server is slow.
SSG (Static Site Generation)
SSG generates HTML during build time. Ideal for content that doesn’t change often, it serves pre-rendered files, making it faster and more cacheable.
When to Use What?
- Use SSR for user-specific or frequently updated content (e.g., dashboards).
- Use SSG for static content like blogs or documentation.
Image Suggestion: A flowchart comparing SSR and SSG, showing how requests are handled for each.
Leveraging Incremental Static Regeneration (ISR)
ISR bridges the gap between SSR and SSG. It allows you to regenerate static pages at runtime, ensuring stale content is updated without rebuilding the entire site.
How to Enable ISR:
export async function getStaticProps(){ const data = await fetchDataFromAPI(); return { props: { data }, revalidate: 60, // Revalidate page every 60 seconds }; }
Best Practices:
- Set a reasonable revalidate interval based on content update frequency.
- Use ISR for blogs, e-commerce product pages, or marketing sites.
Image Suggestion: A diagram showing how ISR works with build-time and runtime updates.
Optimizing Bundle Size
A smaller bundle size ensures faster page loads. Next.js automatically applies tree-shaking, but further optimization is possible.
Steps to Optimize:
- Code Splitting: Split large dependencies into smaller chunks. For instance, dynamically import components where necessary:
const DynamicComponent = dynamic(() => import('./HeavyComponent')); - Analyze Your Bundle: Use Next.js’ built-in bundle analyzer to identify large modules:
- npm install @next/bundle-analyzer
- Add this to your next.config.js:
const withBundleAnalyzer = require('@next/bundle-analyzer')({ enabled: process.env.ANALYZE === 'true', }); module.exports = withBundleAnalyzer({});
- Remove Unused Dependencies: Tools like import cost and Webpack plugins can help identify unused code.
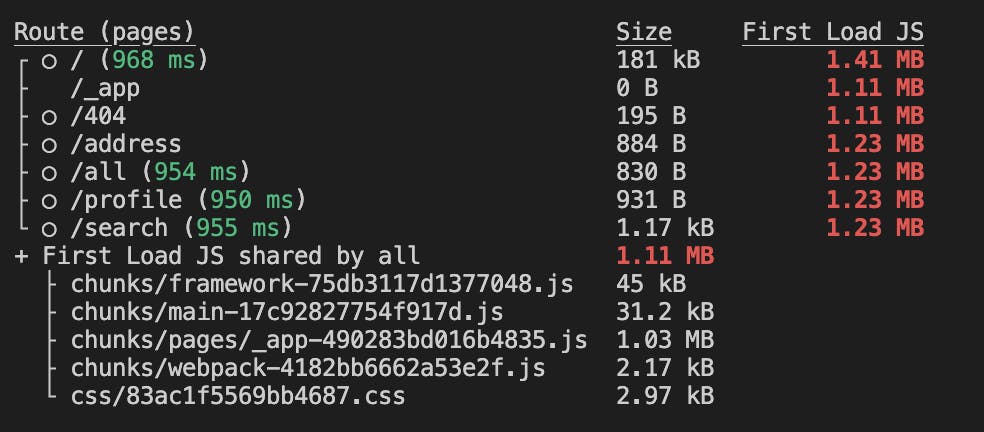
Optimizing Images and Fonts
Image Optimization:
Next.js provides an <Image> component with built-in lazy loading and responsive capabilities. Ensure all images use this for optimization:
import Image from 'next/image'; <Image src="/example.jpg" alt="Example" width={800} height={600} placeholder="blur" />
Tips:
- Use WebP format for smaller file sizes.
- Specify placeholder="blur" for a better user experience.
Font Optimization:
Next.js optimizes Google Fonts automatically. Use the next/font package for modern font management:
import { Inter } from 'next/font/google'; const inter = Inter({ subsets: ['latin'] });
Reducing TTFB (Time to First Byte)
TTFB is the time it takes for the browser to receive the first byte of data from the server. Here's how to minimize it:
1. Use Edge Functions
Deploy server-side logic closer to the user using platforms like Vercel Edge Functions. These functions run globally, reducing latency:
export default async function handler(req, res) { return new Response('Hello from the edge!', { status: 200 }); }
Cache API Responses
Use caching headers to reduce repeated API requests:
res.setHeader('Cache-Control', 's-maxage=10, stale-while-revalidate');
3. Optimize Middleware:
Avoid heavy computation in middleware. Keep it lightweight to prevent delays in request processing.
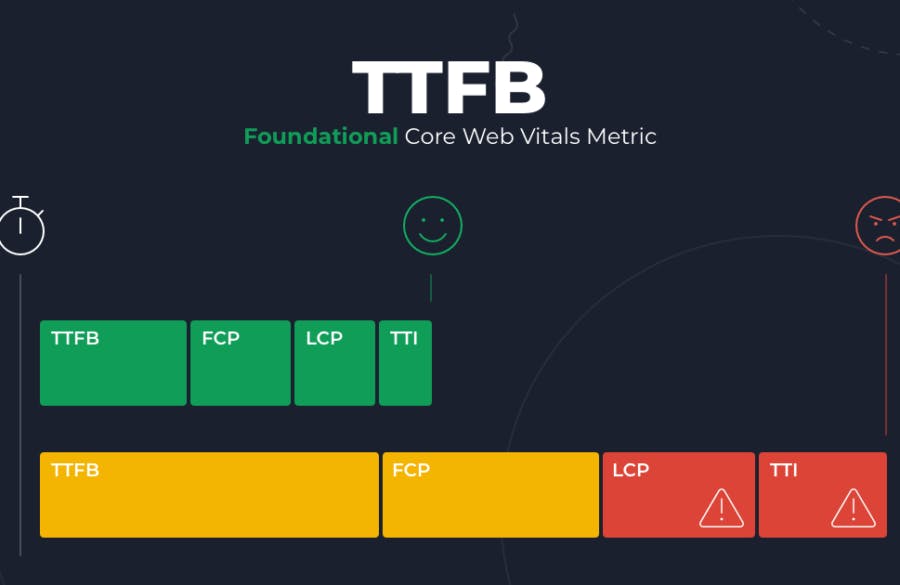
Handling Large Data in API Routes
When working with large datasets in API routes, the following strategies can improve performance:
Pagination:
Break down large datasets into smaller, manageable chunks.
const page = req.query.page || 1; const limit = 10; const data = await fetchLargeData({ page, limit });
Compression:
Compress responses using Gzip or Brotli for faster transmission:
import compression from 'compression'; app.use(compression());
Database Optimization:
Optimize queries to fetch only the required data. Use indexing in MongoDB or MySQL for faster lookups.
Final Thoughts
Optimizing Next.js applications goes beyond default settings—it requires a careful balance of architecture, tools, and techniques. By leveraging features like ISR, optimizing bundles, and reducing TTFB, you can ensure your application delivers blazing-fast performance to users.
What to Read Next?:
- Advanced Caching Strategies in Next.js
- The Ultimate Guide to SSR and CSR
- Real-world Examples of Edge Functions