Exploring WebSockets in Depth: A Technical Guide for Real-Time Applications
Thursday, August 1, 2024
WebSockets have become an essential part of modern web applications, enabling real-time, two-way communication between a client and server. In this guide, we’ll explore how WebSockets work, how they differ from traditional HTTP, and how to implement them effectively in real-world applications.
Understanding WebSockets vs. HTTP
WebSockets are a communication protocol designed to provide full-duplex communication channels over a single, long-lived connection. Unlike the traditional HTTP model, where the client sends a request and waits for a response, WebSockets allow both the client and server to send and receive data at any time.
HTTP
- One-way communication: The client sends a request to the server, and the server responds. After each request, the connection is closed.
- Request-response model: Ideal for static content, but inefficient for real-time communication because of the need for repeated requests (polling).
WebSockets
- Two-way communication: Both the client and server can send data at any time.
- Persistent connection: After the initial handshake, the connection remains open, allowing for low-latency real-time communication.
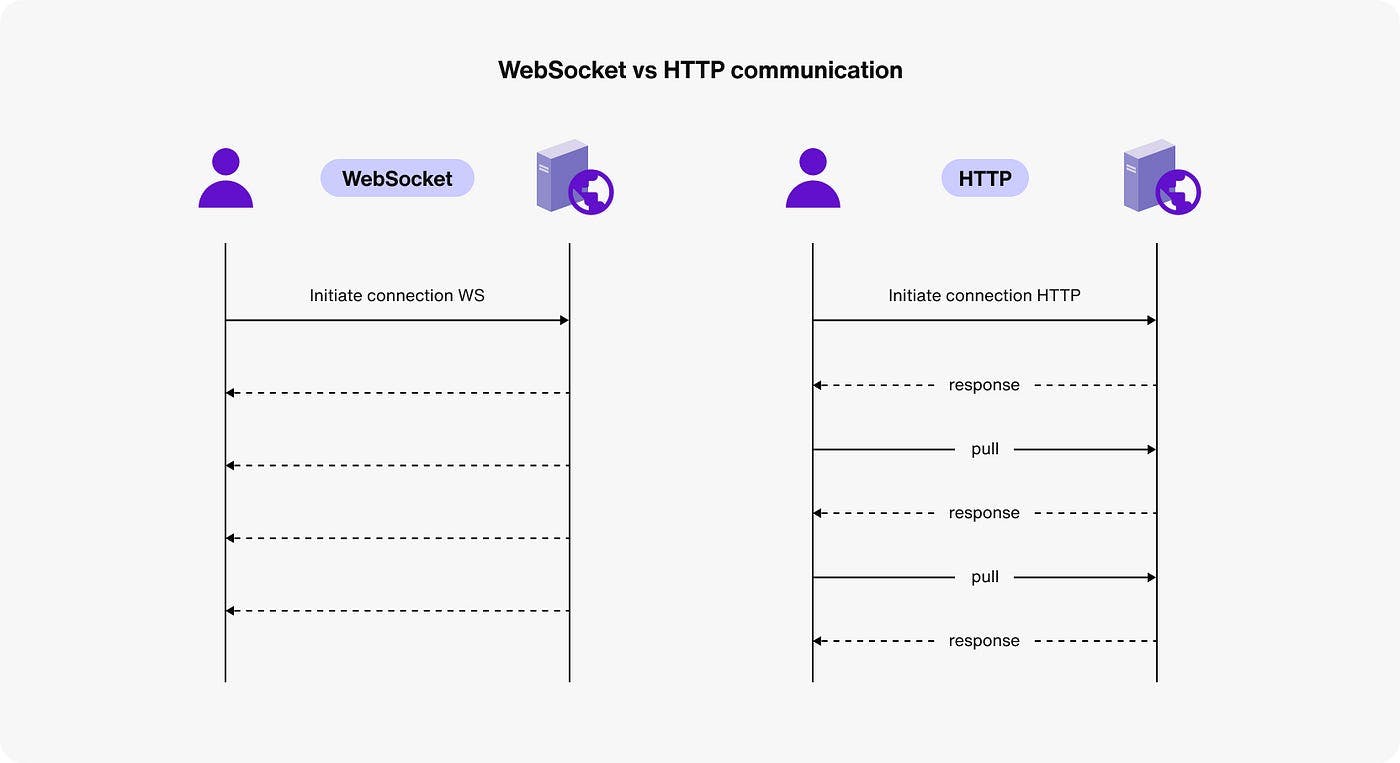
Implementing WebSockets with Node.js and Socket.io
Socket.io is a popular library that simplifies WebSocket implementation in Node.js. It provides automatic reconnection, broadcasting, and event-based communication, which is perfect for real-time applications like chat or notifications.
1. Setting Up the WebSocket Server with Socket.io
To get started, install the socket.io library in your project:
npm install socket.io
Here’s a basic implementation of a WebSocket server using Socket.io:
const { Server } = require("socket.io"); const io = new Server(3000, { cors: { origin: "*", }, }); io.on("connection", (socket) => { console.log("A user connected"); // Listen for messages from clients socket.on("message", (data) => { console.log(data); socket.emit("reply", "Message received!"); }); // Handle disconnections socket.on("disconnect", () => { console.log("A user disconnected"); }); }); console.log("WebSocket server running on http://localhost:3000");
On the client-side, you can use the following code to connect to the WebSocket server:
<script src="https://cdn.socket.io/4.5.0/socket.io.min.js"></script> <script> const socket = io("http://localhost:3000"); socket.on("connect", () => { console.log("Connected to server"); // Send a message to the server socket.emit("message", "Hello, Server!"); // Listen for server responses socket.on("reply", (data) => { console.log(data); }); }); </script>
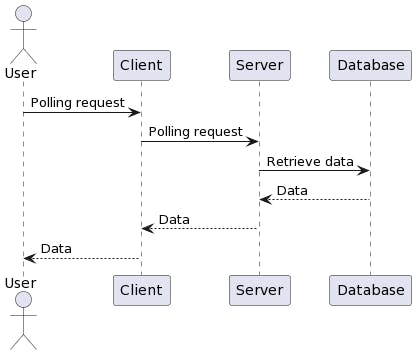
Handling Disconnections and Reconnections
In any real-world application, network failures, server restarts, or other interruptions might disconnect users. WebSocket connections need to be resilient and able to automatically reconnect when these disruptions occur.
Socket.io has built-in mechanisms to handle disconnections and reconnections. By default, Socket.io will attempt to reconnect to the server a few times before giving up.
Customizing Reconnection Behavior
You can customize the reconnection behavior, including the maximum number of attempts and the delay between each attempt:
const io = require("socket.io")(3000, { reconnection: true, // Default is true reconnectionAttempts: 5, // Number of retry attempts before giving up reconnectionDelay: 1000, // Delay in milliseconds between retries });
You can also listen for the reconnect event to track when the client successfully reconnects:
socket.on("reconnect", (attemptNumber) => { console.log(`Reconnected after ${attemptNumber} attempts`); });
And handle the reconnect_failed event when reconnection attempts fail:
socket.on("reconnect_failed", () => { console.log("Failed to reconnect"); });
Load Balancing WebSocket Connections
Scaling WebSocket connections in a distributed system can be challenging because WebSocket connections are persistent, and traditional load balancers are not designed to handle long-lived connections. However, there are several strategies for load balancing WebSocket connections effectively.
Sticky Sessions
One way to ensure a WebSocket connection is routed to the same server after a request is to use sticky sessions. Sticky sessions (also known as session affinity) ensure that all requests from a specific client are sent to the same server instance.
For example, with Nginx, you can configure sticky sessions like this:
upstream websocket_backend { ip_hash; # Ensures that requests from the same client go to the same server server 192.168.1.101; server 192.168.1.102; } server { location /socket.io/ { proxy_pass http://websocket_backend; proxy_http_version 1.1; proxy_set_header Upgrade $http_upgrade; proxy_set_header Connection "upgrade"; } }
Redis for Scaling
For applications with a large number of WebSocket connections, you can use Redis to manage the state across multiple server instances. Socket.io supports Redis adapters that synchronize events between different server instances.
To set up Redis with Socket.io, install the socket.io-redis adapter:
npm install socket.io-redis
Then, configure your WebSocket server to use Redis:
const { createAdapter } = require("@socket.io/redis-adapter"); const { createClient } = require("redis"); const pubClient = createClient({ host: "localhost", port: 6379 }); const subClient = pubClient.duplicate(); io.adapter(createAdapter(pubClient, subClient));
Use Cases for WebSockets
WebSockets are perfect for applications that require real-time, bidirectional communication. Here are a few common use cases:
- Chat Applications: Allow users to send messages instantly without polling the server.
- Live Notifications: Push updates to users in real time, such as notifications for new messages or activities.
- Online Games: Synchronize game state between multiple players in real time.
- Collaborative Tools: Enable multiple users to edit documents or collaborate in real time.
Final notes
WebSockets provide a powerful way to build real-time, interactive web applications. With the ability to send and receive messages instantly, they are ideal for use cases like chat applications, gaming, live notifications, and collaborative tools. By implementing Socket.io in your Node.js applications, handling disconnections, and scaling WebSocket connections with Redis and load balancing, you can create robust, scalable real-time experiences for your users.