Optimizing Server-Side Rendering (SSR) in Next.js 15 for Faster Load Times
Sunday, October 27, 2024
Server-side rendering (SSR) in Next.js offers a powerful way to deliver fast, optimized web experiences, especially when handling dynamic content or SEO-driven pages. With the release of Next.js 15, SSR has been further improved, giving developers more tools to optimize load times and reduce server overhead. This guide will cover key techniques and best practices for optimizing SSR in Next.js 15, focusing on metrics, code strategies, and deployment tips for peak performance.
Understanding SSR and Why It Matters for Performance
In SSR, the server pre-renders a page on each request and sends it as HTML to the browser. This approach differs from client-side rendering (CSR) by delivering faster initial load times and SEO advantages. However, optimizing SSR can significantly reduce server strain, improve time to first byte (TTFB), and enhance the overall user experience.
Key Metrics to Track for SSR Optimization
To monitor and optimize SSR performance effectively, track these essential metrics:
- Time to First Byte (TTFB): The time taken for the server to send the first byte of the response.
- Server-Side Load Time: Time required to generate the HTML on the server.
- Largest Contentful Paint (LCP): Measures how long it takes for the largest element to load, providing insight into perceived load speed.
- Total Blocking Time (TBT): Measures non-interactive time, useful for assessing how SSR might affect interactivity.
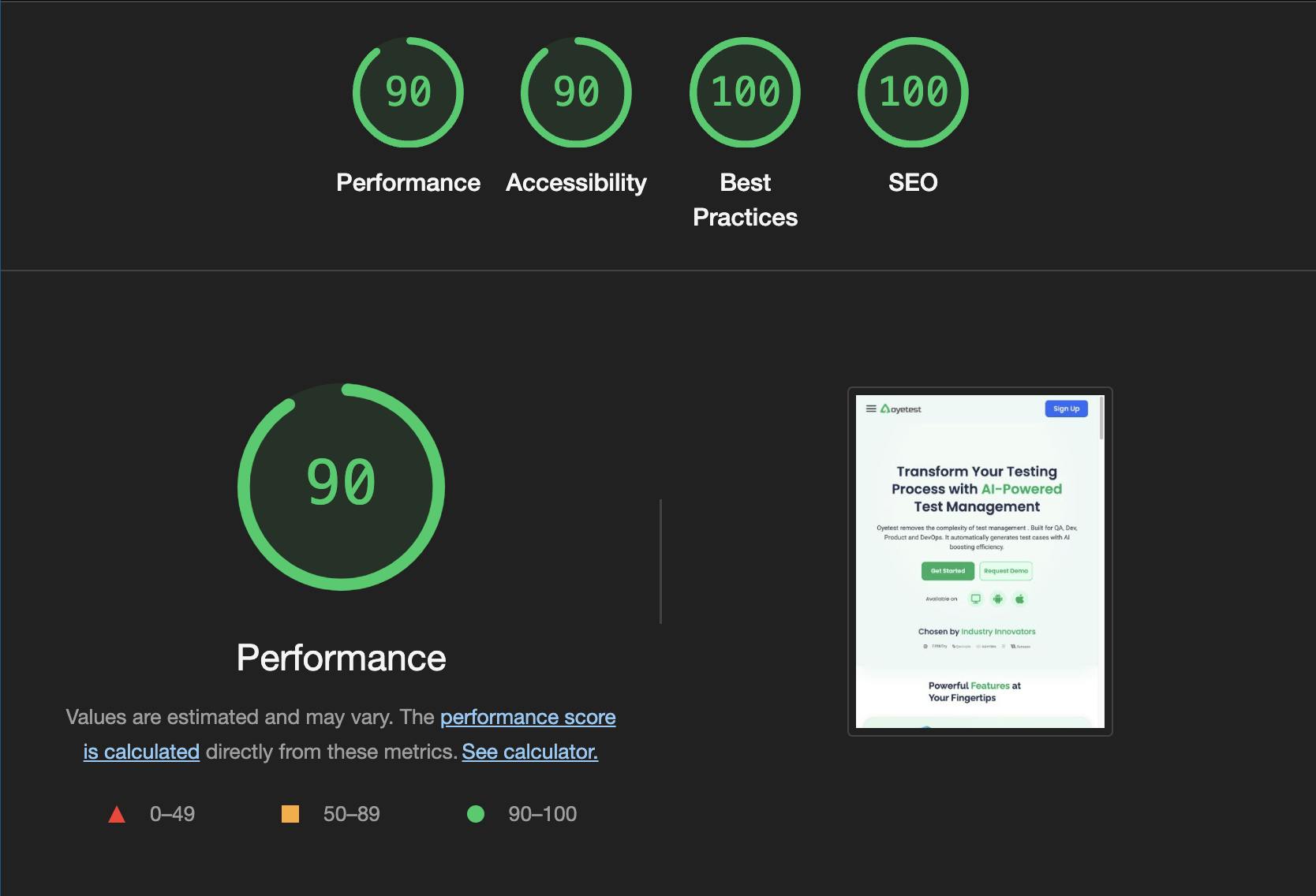
Key Techniques for Optimizing SSR in Next.js 15
1. Use Static Generation (SSG) Where Possible
Static Site Generation (SSG) is faster than SSR for certain types of content. By pre-rendering pages at build time, SSG reduces the load on servers and improves caching capabilities. In Next.js 15, combining SSR and SSG intelligently allows for a hybrid approach.
- Dynamic Static Generation (DSG): For pages that rarely change, use SSG with revalidation (getStaticProps with revalidate) to update content periodically without the overhead of SSR.
// Example of SSG with revalidation for periodic updates export async function getStaticProps() { // Fetch your data here return { props: { data }, revalidate: 60, // Revalidate every 60 seconds }; }
2. Optimize Data Fetching with getServerSideProps
getServerSideProps is crucial for SSR, fetching data at request time and sending it to the client as part of the pre-rendered HTML. To optimize:
- Batch API Calls: Make multiple calls to different endpoints concurrently using Promise.all, reducing overall wait time.
- Memoize Expensive Operations: For computations that don't change often, cache results in memory.
// Using Promise.all to parallelize API calls in `getServerSideProps` export async function getServerSideProps() { const [data1, data2] = await Promise.all([ fetch("https://api.example.com/data1"), fetch("https://api.example.com/data2"), ]); return { props: { data1, data2 } }; }
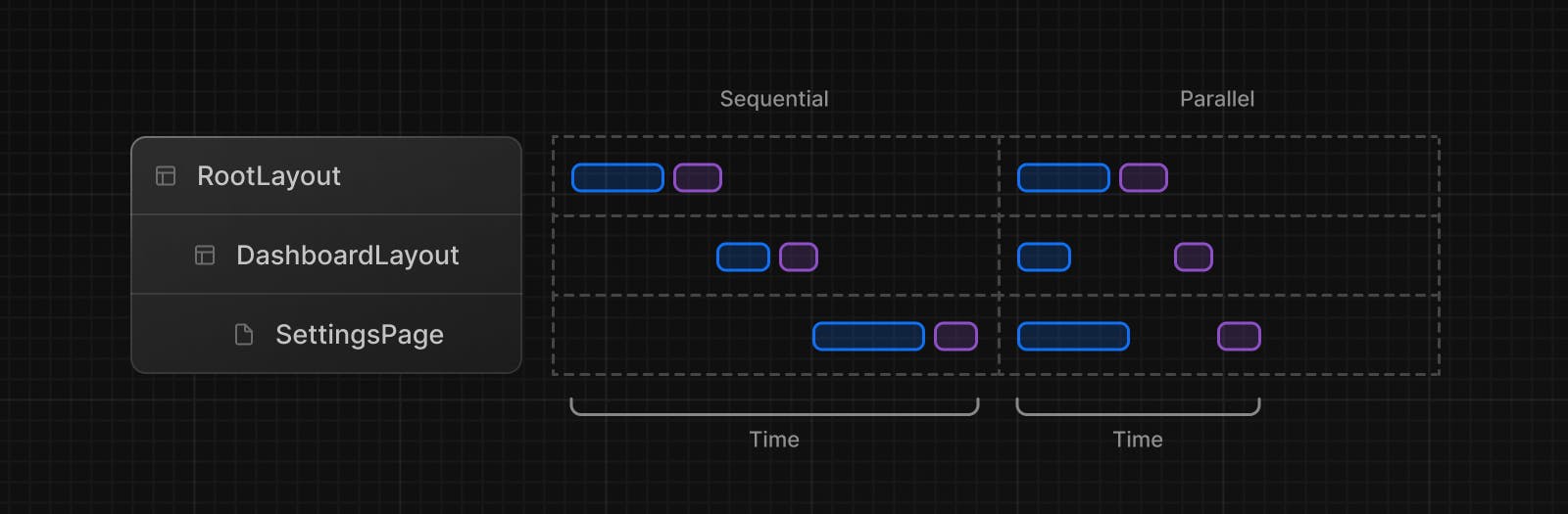
3. Optimize Component Code Splitting
Next.js 15 supports automatic code splitting, but you can control component loading to reduce the JavaScript sent to the client. Use React.lazy or dynamic imports to load components only when needed.
- Dynamic Imports: Use next/dynamic for components that only appear under certain conditions, such as modals or carousels, to avoid loading them with the initial page.
import dynamic from 'next/dynamic'; const DynamicComponent = dynamic(() => import('../components/DynamicComponent'), { ssr: false, });
4. Implement Server-Side Caching Strategies
Caching is a powerful way to boost SSR performance by serving previously rendered HTML instead of generating it from scratch. Next.js offers several approaches to caching:
- HTTP Caching Headers: Set headers in getServerSideProps to specify cache duration, e.g., Cache-Control.
- CDN Caching: Use a content delivery network (CDN) like Vercel or Cloudflare to cache content at the edge.
// Setting HTTP headers in `getServerSideProps` export async function getServerSideProps({ res }) { res.setHeader('Cache-Control', 'public, s-maxage=10, stale-while-revalidate=59'); return { props: {} }; }
5. Prefetch Data Efficiently with useSWR for Partial SSR
Next.js 15 introduces smoother client-side data fetching capabilities. You can use useSWR for client-side revalidation with partial SSR to reduce server load while keeping data fresh.
import useSWR from 'swr'; function ExampleComponent() { const { data, error } = useSWR('/api/data', fetcher); if (error) return <div>Failed to load</div>; if (!data) return <div>Loading...</div>; return <div>{data}</div>; }
6. Use Edge Functions for Faster Data Fetching
For low-latency applications, edge functions allow you to run server logic close to the user, reducing TTFB. Edge functions in Vercel can cache responses and offer better control over SSR content served at the edge.
Testing and Measuring SSR Performance
After implementing optimizations, test performance regularly. Use Google Lighthouse for a comprehensive audit and track metrics over time. WebPageTest offers detailed insights, allowing you to visualize load times, TTFB, and LCP for specific locations.
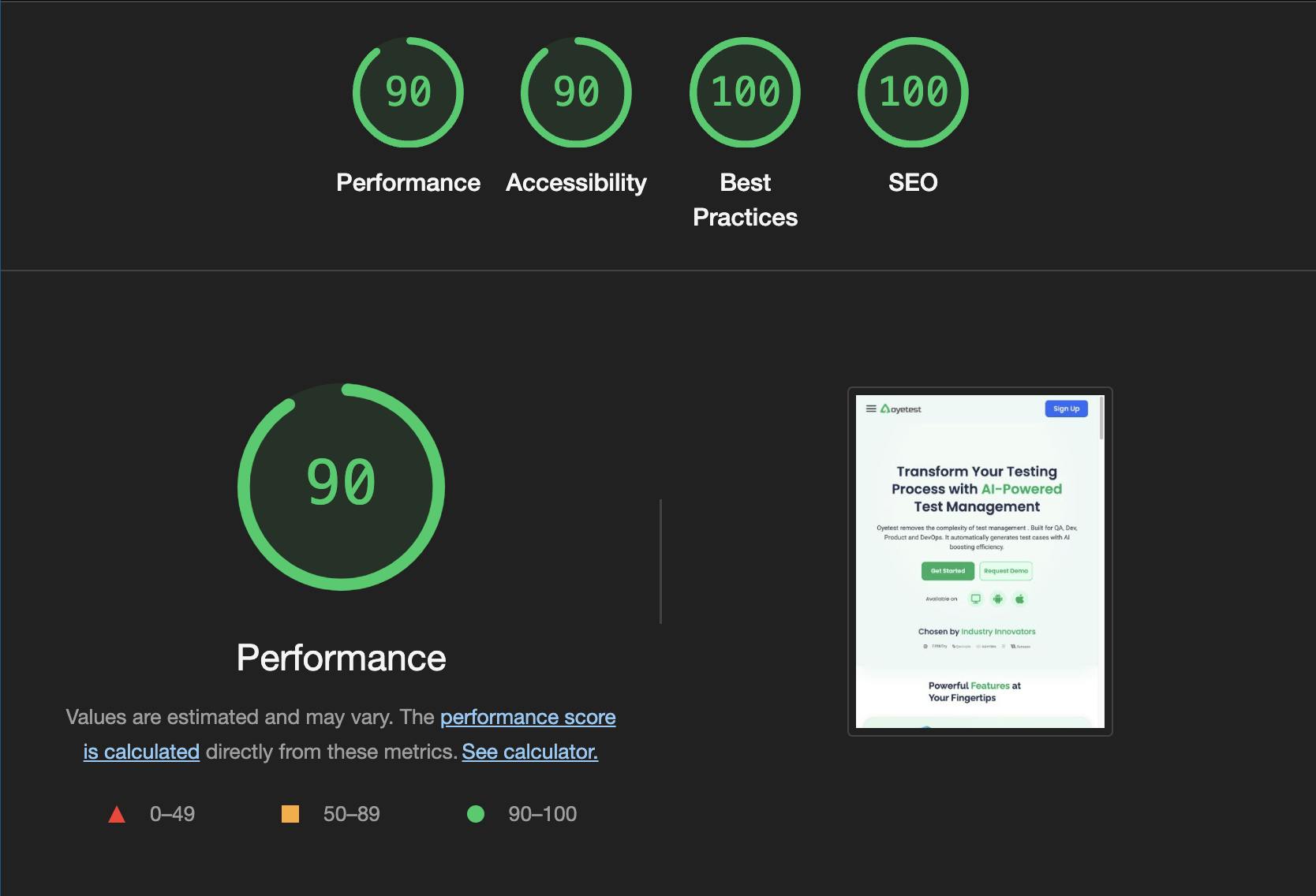
By implementing these optimization techniques, Next.js 15 applications can achieve significant performance improvements in server-side rendering. Utilizing SSR strategically with caching, code splitting, and efficient data fetching ensures faster load times, reduced server load, and an enhanced user experience.
Explore more about SSR in the Next.js Documentation : https://nextjs.org/docs/app/building-your-application/rendering/server-components
Author
Saurabh Rai